Internal API Call
Internal API Call allows users to invoke an internal call based on the settings defined.
Configuring the interceptor
The images and text below present the information that must be informed to configure the interceptor.
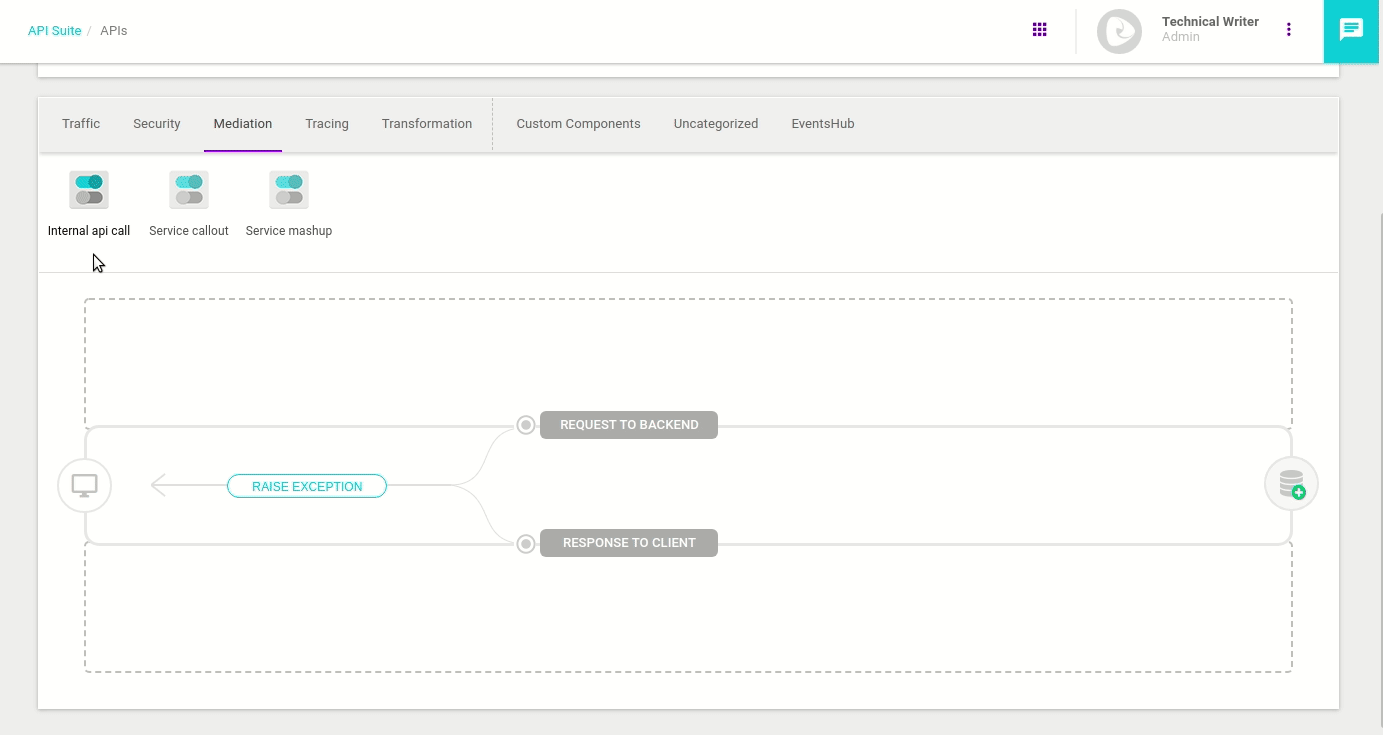
-
Variable Name: defines the name of the Internal API Call, unique per flow. The call response is retrieved from the map
$call.contextVariables
using the name informed here. A custom interceptor is required for this, as shown below. -
API: API to be invoked.
-
Revision: revision of the API to be invoked.
-
Resource: resource to be invoked.
-
Operation: operation to be performed.
You can only invoke an API that is deployed to the same environment as the API to whose flow you are adding the interceptor. Going back to the APIs in the example of use above, to call the API "AuthenticateUser", it must be deployed to the same environment as the API "SearchSalesInformation", which is the one that contains the Internal API Call interceptor. If you wish to invoke an API that is not deployed to the same environment, you must first deploy it. Remember that you can deploy an API to multiple environments. You can read more about deploying APIs here. |
-
Preserve Query String: if checked, the query string present in the request will be transferred to the API invoked internally.
-
Query: allows the insertion of a query string.
-
Preserve Path Param: if checked, the path param present in the request will be transferred to the API invoked internally.
-
Path Param: allows the insertion of a path param.
-
Preserve Body: if checked, the body contained in the request will be transferred to the API invoked internally. This field is enabled when the method is different from GET.
-
Body: allows the insertion of a body. The field is enabled when the method is different from GET and the field Preserve Body is unchecked.
-
Preserve Headers: if checked, the headers present in the request will be transferred to the API invoked internally.
-
Headers: allows the insertion of headers.
How it works
To show how Internal API Call works, let’s consider the following case:
In the case of internal communication, there is already an exposed API that sends the message through the internal system (radio-messages) and aggregates the responses: the LSA Internal Communication. To take advantage of this API, already deployed in the same environment as LSA Communication, we use an Internal API Call.
The following images show, respectively, the sequence of interceptors in the API flow and the configurations used in the Internal API Call:
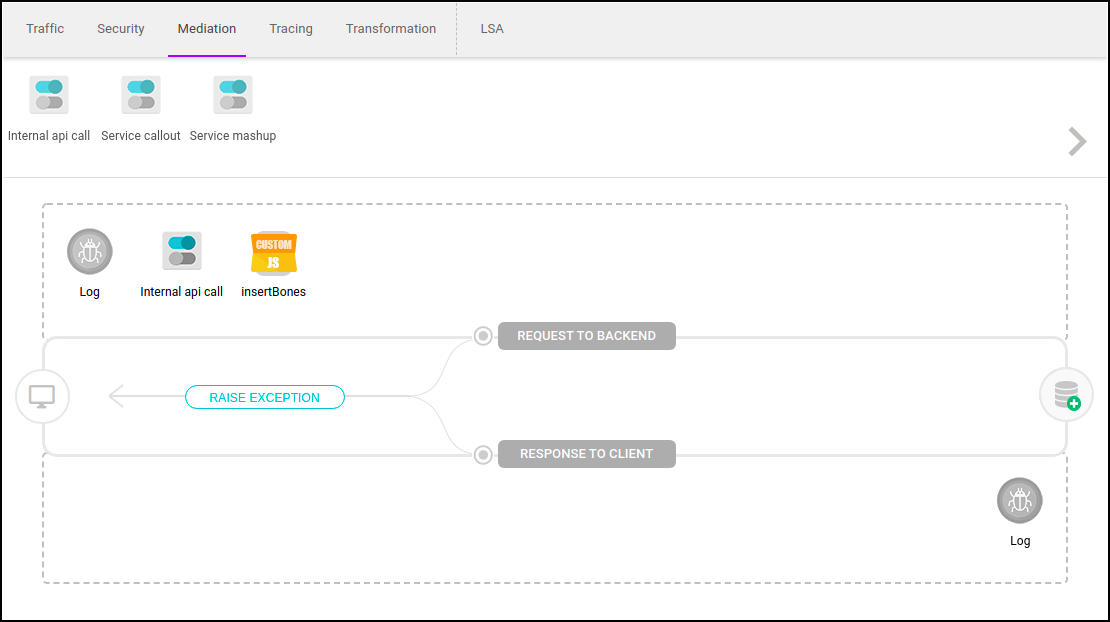
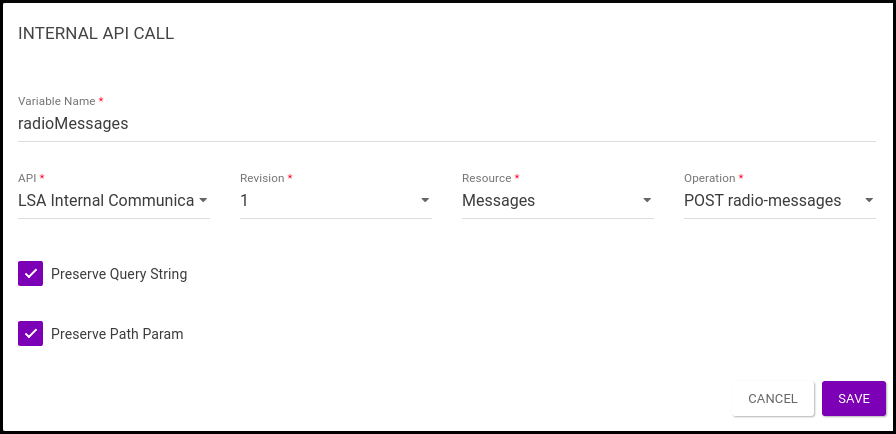
LSA Internal Communication returns, with status code 200, the following body[1]:
{
"messages": [
{
"message": "I've already joined, Sal, a year ago. Is this just a test message?",
"author": "Eva"
}
]
}
After the call, the interceptor will return an object of type ApiResponse that will be stored in the call’s context variables.
To retrieve the response, you need to create a custom interceptor that accesses $call.contextVariables.get(“Variable Name”)
.
Below is the code for the custom JavaScript interceptor that will be used to capture the response and include the body and status in the final response:
// Capture the response from Internal API Call
var respRadio = $call.contextVariables.get('radioMessages');
// Create an ApiResponse Object for this call
$call.response = new com.sensedia.interceptor.externaljar.dto.ApiResponse();
$call.response.addHeader('Content-Type', 'application/json');
// JSON template for returned messages
var respBody = JSON.parse('{"radio": {"status": 0, "body": {}}}');
// The status returned is an Object, not a Number
var statusRadio = respRadio.getStatus();
// The intValue method is called to get a Number object
respBody.radio.status = statusRadio.intValue();
// A body is inserted only if a 200 status is returned
if (statusRadio == 200){
respBody.radio.body = JSON.parse(respRadio.getBody().getString("UTF-8"));
}
// Set the modified body into the ApiResponse
$call.response.getBody().setString(JSON.stringify(respBody), "UTF-8");
// Set return status as 200
$call.response.setStatus(200);
Finally, the body of the response of the POST call to the internal-messages resource of LSA Communication will be:
{
"radio": {
"status": 200,
"body": {
"messages": [
{
"message": "I've already joined, Sal, a year ago. Is this just a test message?",
"author": "Eva"
}
]
}
}
}
Share your suggestions with us!
Click here and then [+ Submit idea]