Service Callout
As with the Internal API Call interceptor, Service Callout allows an API to invoke a call based on pre-defined settings. The difference is that, while the former redirects a call to APIs that are available in the Sensedia Platform, Service Callout allows invoking an external call.
Configuring the interceptor
The images and text below present the information that must be informed to configure the interceptor.
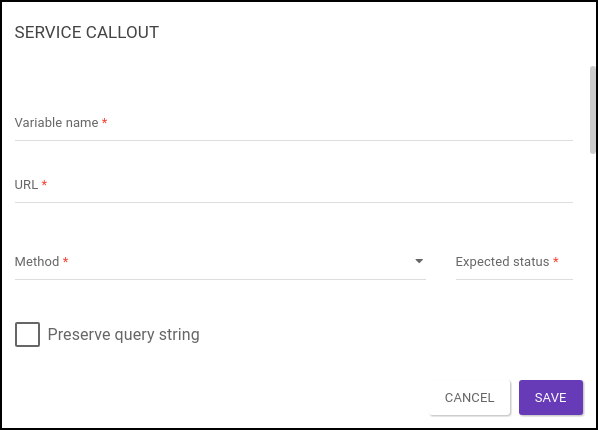
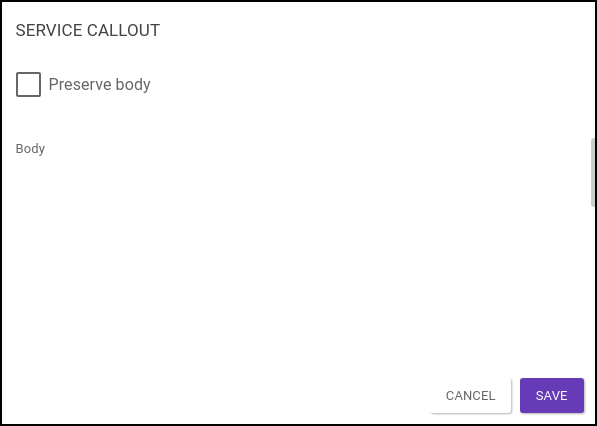
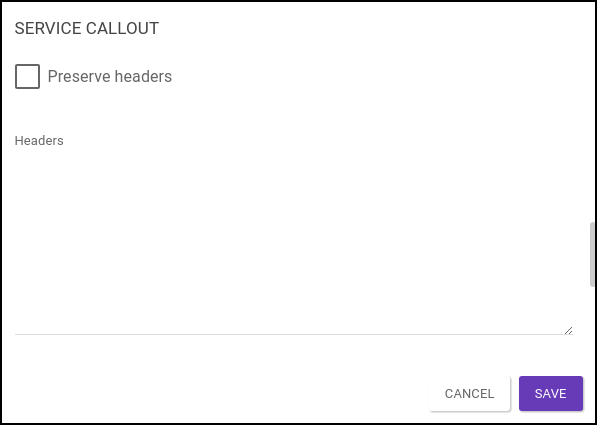
-
Variable name: it defines the name of the service callout, being unique per flow. The call response is retrieved from the map
$call.contextVariables
using the name informed here. A custom interceptor is required for this, as shown below. -
URL: it defines the URL that will be invoked in the external request.
-
Method: it defines the HTTP method to be used;
-
Expected status: it sets the expected status code.
-
Preserve query string: if checked, the query string present in the request will be passed on to the external call.
-
Preserve body: if checked, the body contained in the request will be passed on to the external call. The field is enabled when the method is different from GET.
-
Body: it allows the insertion of a body. The field is enabled when the method is different from GET and the field Preserve body is unchecked.
-
At this point, it is possible to use environment variables, however, it is not possible to use more than one. For more information on environment variables, click here.
-
-
Preserve headers: if checked, the headers present in the request will be passed on to the external call.
-
Headers: it allows the insertion of headers.
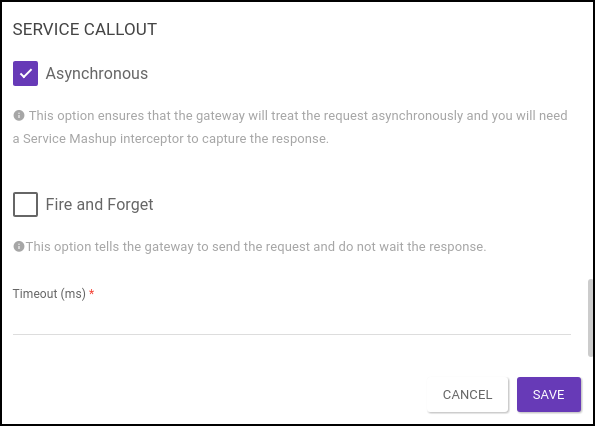
Service Callout has the ability to make synchronous or asynchronous calls. In the latter case, a synchronous call will be sent to the new URL and the interceptor will await the result to continue the execution of the flow. For asynchronous calls, select the field Asynchronous.
The interceptor Service Mashup must be inserted in the API flow to allow capturing the response of asynchronous calls. |
You can select the option Fire and Forget or leave it unchecked. If you select it, the interceptor will send the request and forget it, meaning that there will be no return from the external call.
When the option is not selected, you can configure a time limit for the external call to be made. The call will be terminated if the timeout reaches its limit or a result is returned.
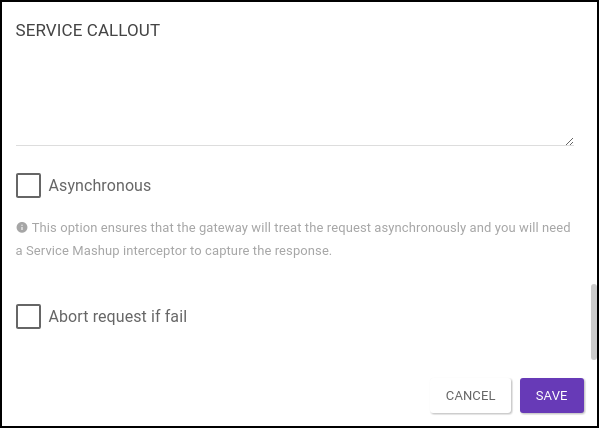
-
Abort request if fail: it aborts the process if the request fails. A failure occurs when the status code of the request is different from the expected. The field is enabled when the request is synchronous.
Error status codes
The status codes returned by Service Callout when an error occurs, and the reasons for them, are:
-
417: if the expected status is different from the status returned by the call.
-
408: if the call exceeds the time limit (timeout).
-
500: any case other than those mentioned.
How it works
To show how Service Callout works, let’s consider the following case:
In the case of external communication, there is an API exposed, under the domain lsa-spies.org.mx and external to the Sensedia’s platform, which allows sending the message through the external system (pigeons). This system has two resources for this: pigeons/pigeon-manny and pigeons/pigeon-meche. To take advantage of this API, we use Service Callout.
Since they are different resources, two Service Callout interceptors will be used. The following images show, respectively, the sequence of the interceptors in the API flow and the settings used in the interceptor that makes the POST call to pigeons/pigeon-manny, being similar the settings for pigeons/pigeon-meche:
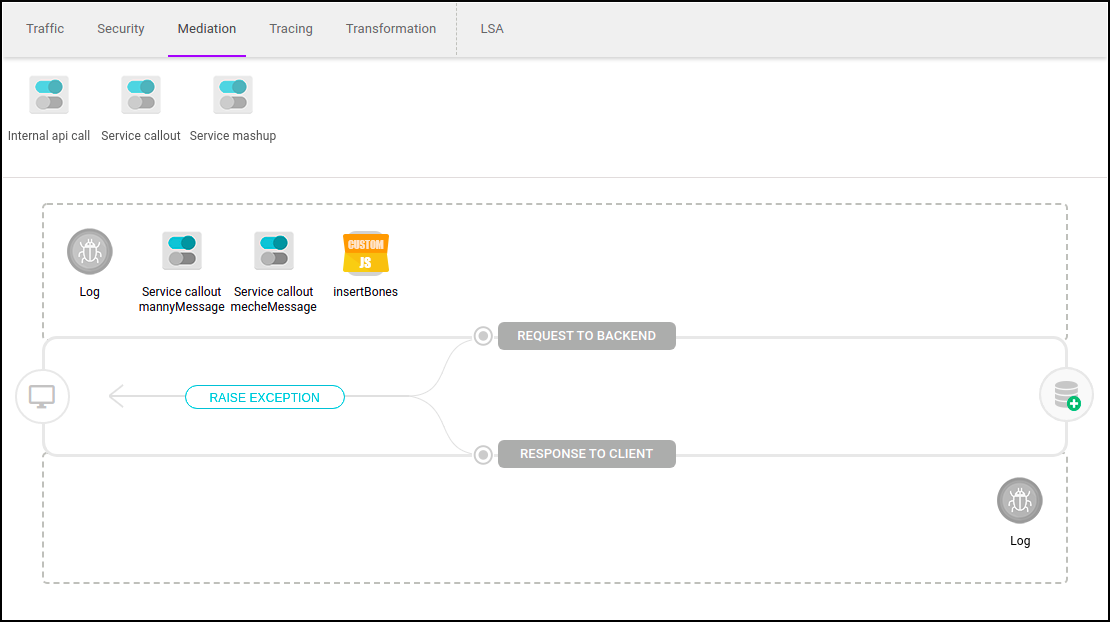
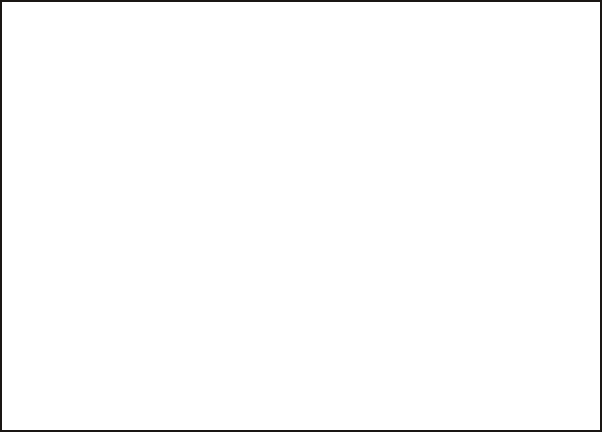
It is important to point out that, because they have been configured to work synchronously, the second Service Callout interceptor will only be executed after the first one has finished executing. When execution time is a relevant issue, it is recommended to use asynchronous calls, as exemplified in the use of Service Mashup.
The call to pigeons/pigeon-manny returns, with status code 200, the following body:
{
"message": "I'm already dead...",
"author": "Manuel Calavera"
}
On the other hand, the call to pigeons/pigeon-meche returns, also with status code 200, the following body:
{
"message": "Only if it has cars, as I was given one purpose, one skill, one desire: to DRIVE!",
"author": "Glottis"
}
After each synchronous call, the interceptor will return an object of type RESTResponse that will be stored in the call’s context variables.
To retrieve the response, you need to create a custom interceptor that accesses $call.contextVariables.get(“Variable Name”)
.
Below is the code for the custom JavaScript interceptor that will be used to capture the responses and include the bodies and status in the final response:
// Capture the responses from Service Callouts
var respManny = $call.contextVariables.get('mannyMessage');
var respMeche = $call.contextVariables.get('mecheMessage');
// Create an ApiResponse Object for this call
$call.response = new com.sensedia.interceptor.externaljar.dto.ApiResponse();
$call.response.addHeader('Content-Type', 'application/json');
// JSON template for returned messages
var respBody = JSON.parse(`{
"pigeonManny": {"status": 0, "body": {}},
"pigeonMeche": {"status": 0, "body": {}}}
`);
// The status returned is an Object, not a Number
var statusManny = respManny.getStatus();
var statusMeche = respMeche.getStatus();
// The intValue method is called to get a Number object
respBody.pigeonManny.status = statusManny.intValue();
respBody.pigeonMeche.status = statusMeche.intValue();
// A body is inserted only if a 200 status is returned
if (statusManny == 200){
respBody.pigeonManny.body = JSON.parse(respManny.getResponseText());
}
if (statusMeche == 200){
respBody.pigeonMeche.body = JSON.parse(respMeche.getResponseText());
}
// Set the modified body into the ApiResponse
$call.response.getBody().setString(JSON.stringify(respBody), "UTF-8");
// Set return status as 200
$call.response.setStatus(200);
Finally, the body of the response of the POST call to the external-messages resource of LSA Communication will be:
{
"pigeonManny": {
"status": 200,
"body": {
"message": "I'm already dead...",
"author": "Manuel Calavera"
}
},
"pigeonMeche": {
"status": 200,
"body": {
"message": "Only if it has cars, as I was given one purpose, one skill, one desire: to DRIVE!",
"author": "Glottis"
}
}
}
Share your suggestions with us!
Click here and then [+ Submit idea]